Python字符提取方法详解
- 使用字符串方法提取符号
在Python中,提取字符串中的特定符号可以通过多种方式实现。以下是一些常用的方法:
str.find()
方法:查找子字符串的位置。

- 示例代码:
```python
text "Hello, world!"
symbol "o"

position text.find(symbol)
if position ! -1:
print(f"Symbol '{symbol}' found at position {position}.")
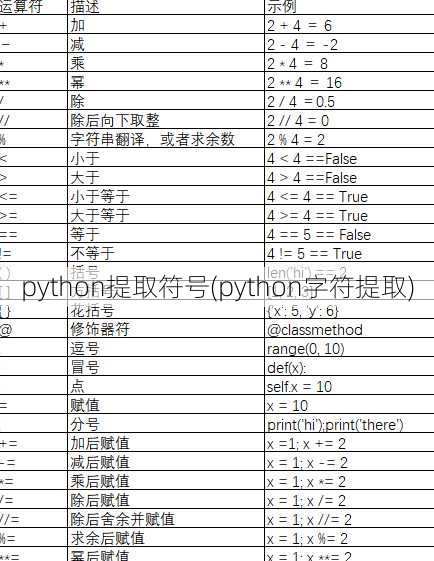
else:
print(f"Symbol '{symbol}' not found.")
```
str.replace()
方法:替换字符串中的特定符号。
- 示例代码:
```python
text "Hello, world!"
symboltoreplace "o"
replacement "0"
newtext text.replace(symbolto_replace, replacement)
print(new_text)
```
str.split()
方法:根据特定符号分割字符串。
- 示例代码:
```python
text "Hello, world!"
symboltosplit ","
parts text.split(symboltosplit)
print(parts)
```
- 使用正则表达式提取符号
正则表达式是Python中处理字符串的强大工具,可以用来提取符合特定模式的字符。
re.findall()
方法:查找所有符合正则表达式的子字符串。
- 示例代码:
```python
import re
text "Hello, world! 2023"
pattern r"d+"
matches re.findall(pattern, text)
print(matches) 输出:['2023']
```
re.search()
方法:搜索第一个符合正则表达式的子字符串。
- 示例代码:
```python
import re
text "Hello, world! 2023"
pattern r"d+"
match re.search(pattern, text)
if match:
print(f"Found: {match.group()}")
else:
print("No match found.")
```
FAQs
Q1:如何提取一个字符串中的所有数字?
A1.1:使用
str.isalnum()
方法示例代码:
```python
text "abc123def456"
numbers ''.join([char for char in text if char.isalnum() and char.isdigit()])
print(numbers) 输出:'123456'
```
A1.2:使用正则表达式
示例代码:
```python
import re
text "abc123def456"
pattern r"d+"
numbers re.findall(pattern, text)
print(''.join(numbers)) 输出:'123456'
```
A1.3:使用字符串方法
示例代码:
```python
text "abc123def456"
numbers ''.join([char for char in text if char.isdigit()])
print(numbers) 输出:'123456'
```
Q2:如何提取一个字符串中的所有非字母字符?
A2.1:使用正则表达式
示例代码:
```python
import re
text "Hello, world! 2023"
pattern r"[^a-zA-Z]"
nonalphachars re.findall(pattern, text)
print(''.join(nonalphachars)) 输出:', ! 2023'
```
A2.2:使用字符串方法
示例代码:
```python
text "Hello, world! 2023"
nonalphachars ''.join([char for char in text if not char.isalpha()])
print(nonalphachars) 输出:', ! 2023'
```
A2.3:使用列表推导式
示例代码:
```python
text "Hello, world! 2023"
nonalphachars [char for char in text if not char.isalpha()]
print(''.join(nonalphachars)) 输出:', ! 2023'
```
Q3:如何提取一个字符串中的所有空格?
A3.1:使用字符串方法
示例代码:
```python
text "Hello, world! 2023"
spaces ''.join([char for char in text if char.isspace()])
print(spaces) 输出:' '
```
A3.2:使用正则表达式
示例代码:
```python
import re
text "Hello, world! 2023"
pattern r"s"
spaces re.findall(pattern, text)
print(''.join(spaces)) 输出:' '
```
A3.3:使用列表推导式
示例代码:
```python
text "Hello, world! 2023"
spaces [char for char in text if char.isspace()]
print(''.join(spaces)) 输出:' '
```